When writing .NET code to access a SQL database we often rely on the Entity Framework (EF). The EF makes it very easy to retrieve data from the database by generating a SQL Query for us. But we should not trust it blindly, as the EF can also generate a bad query.
It will return the correct data yes, but at what performance cost?
I have a table with lots of data, and to keep the table as small as possible on disk and in memory I want to optimize the columns. This can be done by using varchar instead of nvarchar, date instead of datetime and using tinyint instead of int. Of course, you only can do this if your data allows this.
The problem
Changing a column in my table from int
to tinyint
gave me a storage and memory space win, but it also gave me a performance loss!
In my case the SQL column HasSong
is a tinyint
.
EF translates this to a Byte structure in the .NET model.
The following code:
var query = from d in dataEntities.Donations where d.HasSong == 1 select d.DonationId;
produces this SQL Query:
SELECT [Extent1].[DonationId] AS [DonationId]FROM [dbo].[Donations] AS [Extent1]WHERE 1 = CAST([Extent1].[HasSong] AS int)
When SQL encounters this CAST it will skip all Indexes that are on the HasSong
column resulting in the query engine using non-optimized indexes or even worse: full table scans.
So, this explains my performance loss, but how do we convince EF not to cast my byte
to an int
?
The familiar “Contains”
Browsing the internet gave me my first idea: using the familiar contains method I encountered on an earlier post about the EF: Joining an IQueryable with an IEnumerable.
I just have to add an Array with a single value.
So, trying this:
var tinyintComparison = new byte[] { 1 };
var query = from d in dataEntities.Donations where tinyintComparison.Contains(d.HasSong) selectd.DonationId;
was not such a good idea as it throws an ArgumentException
:
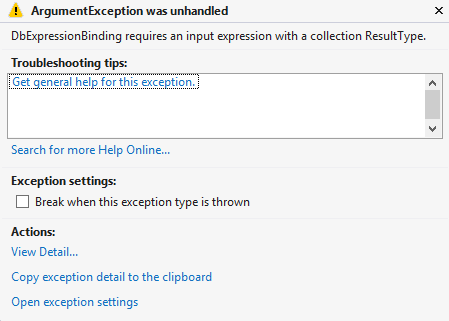
It did work for other people using a SMALLINT, so I guess an array of bytes is a special case reserved for working with binary data in your database.
The solution
Lucky enough we are close to a solution, changing the array to a List<T>
class:
var tinyintComparison = new List<byte> { 1 };
var query = from d in dataEntities.Donations where tinyintComparison.Contains(d.HasSong) select d.DonationId;
results in the following query:
SELECT [Extent1].[DonationId] AS [DonationId]FROM [dbo].[Donations] AS [Extent1]WHERE 1 = [Extent1].[HasSong]
There we are! No more casting. The SQL query engine can use the correct indexes again and we still won disk and memory space.
Conclusion
If you don’t want a CAST in your SQL query when using a tinyint
, use the
ICollection<T>.Contains
method.